JBoss Drools Hello World-Stateful Knowledge Session using KieSession
JBoss Drools - Table of Contents
JBoss Drools Hello World JBoss Drools Hello World-Stateful Knowledge Session using KieSession JBoss Drools- Understanding Drools Decision Table using Simple Example Understand Drools Stateful vs Stateless Knowledge Session Drools Tutorials- Backward Chaining simple example Drools Tutorials- Understanding attributes salience, update statement and no-loop using Simple Example Drools Tutorials- Understanding Execution Control in Drools using Simple Example Drools Tutorials- Integration with Spring MVC Drools Tutorials- Integration with Spring Boot
Overview
In previous chapter we implemented a simple drools project to execute simple rule. From Drools 6.0 onwards a new approach is used to create a Knowledge Base and a Knowledge Session. Knowledge base is an interface that manages a set of rules and processes. The main task of the knowledge base is to store and re-use rules because creation of rules is very expensive.Rules are contained inside the package org.drools.KnowledgeBase. These are commonly referred to as knowledge definitions or knowledge. Knowledge base provides methods for creating a Session.The knowledge session can be of two types:
- Stateless Knowledge Session
- Stateful Knowledge Session
Video
This tutorial is explained in the below Youtube Video.Lets Begin
Using drools we have to define the discounts offered on various jewellery products depending on the type. For example if the product is jewellery item offer a discount of 25% and so on. We will create Eclipse Maven project as follows-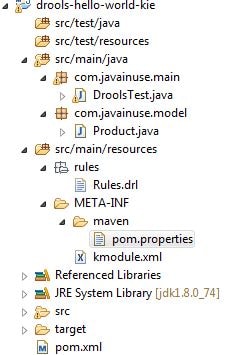
The POM defined is as follows- Only a single dependency og Drools-compiler is required.
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <groupId>com.javainuse</groupId> <artifactId>drools-hello-world</artifactId> <version>0.0.1-SNAPSHOT</version> <properties> <drools.version>6.2.0.Final</drools.version> </properties> <dependencies> <dependency> <groupId>org.kie</groupId> <artifactId>kie-api</artifactId> <version></version> </dependency> <dependency> <groupId>org.drools</groupId> <artifactId>drools-core</artifactId> <version></version> </dependency> <dependency> <groupId>org.drools</groupId> <artifactId>drools-compiler</artifactId> <version></version> </dependency> <dependency> <groupId>org.kie</groupId> <artifactId>kie-ci</artifactId> <version></version> </dependency> </dependencies> </project>Next define the Model class Product which defines the type of jewellery item.
package com.javainuse.model; public class Product { private String type; private int discount; public String getType() { return type; } public void setType(String type) { this.type = type; } public int getDiscount() { return discount; } public void setDiscount(int discount) { this.discount = discount; } }